Java programming: Java program consists of instructions that will be executed on a machine to perform a task. For example, say arrange given integers in ascending order. This page contains programs for beginners to understand how to use Java programming to write simple Java programs. These programs show how to get input from a user, working with loops, strings, and arrays. Don't forget to see a program output (image file), and you can also download the class file and execute it directly without compiling the source file.
Compiling and executing Java programs
Java programming software: To compile and run Java program you need to download JDK (Java Development Kit).
To compile type: javac file_name.java where file_name is the name of the file containing Java source code.
Javac is the Java compiler which converts java code into bytecode.
To run type: java MainMethodClass where MainMethodClass is the name of the class which defines the "main" method.
Learn Java through books
If you are just starting to learn Java, then it's recommended to buy Java programming book. It will help you to learn basic concepts and will be a reference when required.
Java programming examples
Example 1: Display message on computer screen.
class First {
public static void main(String[] arguments) {
System.out.println("Let's do something using Java technology.");
}
}
This is similar to a hello world Java program.
Download java programming class file.
Output of program:
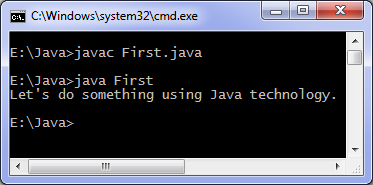
Example 2: Print integers
class Integers {
public static void main(String[] arguments) {
int c; //declaring a variable
/* Using a for loop to repeat instruction execution */
for (c = 1; c
Output:
<img src="/images/java/integers-java-program.png" alt="10 natural numbers java program" />
If else control instructions:
<java>
class Condition {
public static void main(String[] args) {
boolean learning = true;
if (learning) {
System.out.println("Java programmer");
}
else {
System.out.println("What are you doing here?");
}
}
}
Output:
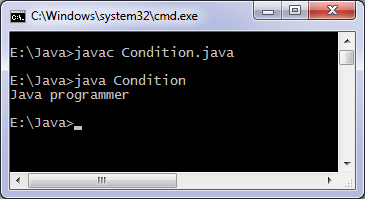
Command line arguments:
class Arguments {
public static void main(String[] args) {
for (String t: args) {
System.out.println(t);
}
}
}
Java programming language
Below is the list of java programs which will help you in learn java programming language.
- Hello World
- If else
- For loop
- While loop
- Print Alphabets
- Print Multiplication Table
- Get Input From User
- Addition
- Find Odd or Even
- Fahrenheit to celsius
- Java Methods
- Static Block
- Static Method
- Multiple classes
- Java constructor tutorial
- Java exception handling tutorial
- Swapping
- Largest of three integers
- Enhanced for loop
- Factorial
- Primes
- Armstrong number
- Floyd's triangle
- Reverse String
- Palindrome
- Interface
- Compare Strings
- Linear Search
- Binary Search
- Substrings of string
- Display date and time
- Random numbers
- Garbage Collection
- IP Address
- Reverse number
- Add Matrices
- Transpose Matrix
- Multiply Matrices
- Bubble sort
- Open notepad
Java Development IDE
As your programming experience grows in Java you can create your project or software, using a simple text editor isn't recommended. Two popular open source IDE's are:
Using IDE helps you a lot while coding, it offers many useful features. You can create GUI in Netbeans without writing any code, Netbeans will show you any compilation error before you compile your code and it also shows hints on how to fix that.
Java programming tutorial
Java technology has changed our life as most of the devices we use today uses it, that's why to learn Java programming is a good thing. It was developed by Sun Microsystems but is now owned by Oracle. Here is a quick Java tutorial, it's an object-oriented computer programming language like C++ if you're familiar with it or any other object-oriented programming language, then it will be easier for you to learn it. A Java program consists of classes that contain methods; you can't write a method outside of a class. Objects are instances of classes. Consider the following program:
class ProgrammingLanguage {
//attributes
String language_name;
String language_type;
//constructor
ProgrammingLanguage(String n, String t) {
language_name = n;
language_type = t;
}
//main method
public static void main(String[] args) {
//creating objects of class
ProgrammingLanguage C = new ProgrammingLanguage("C", "Procedural");
ProgrammingLanguage Cpp = new ProgrammingLanguage("C++", "Object oriented");
//calling method
C.display();
Cpp.display();
}
//method (function in C++ programming)
void display() {
System.out.println("Language name:" + language_name);
System.out.println("Language type:" + language_type);
}
}
There is a ProgrammingLanguage class, and all programming languages are instances of this class. The class has two attributes language name and type; we can create instances of the class using "new" keyword. The constructor method (has the same name as that of the class) is invoked when an object of the class is created; we use it to name the programming language and its type. "Main" method is a must and acts as a starting point of a program, the "display" method is used to print information about the class object. A class name begins with a capital letter, and if there are more words in the class name, then their first letters are also capital. For example, MyJavaClass is a class name, and for methods (functions in C/C++), the first letter is small and in other words, the first letter is capital, for example, myJava is method name. These are only conventions but are useful in distinguishing classes from methods. Java has a very rich API to build desktop and web applications.
Java programming PDF
If you like to learn through a PDF, then try following e-books:
Java beginner's guide.
Introduction to programming using Java.